1. Queue
์ ์ ์ ์ถ ํ๋ ๋ฉํฐ ํ์คํน์ ์ํ ํ๋ก์ธ์ค ์ค์ผ์ค๋ง ๋ฐฉ์์ ๊ตฌํํ๊ธฐ ์ํด ๋ง์ด ์ฌ์ฉ๋๋ค.(์ด์์ฒด์ )
์๋ฐ์์๋ ํ์ ๊ฒฝ์ฐ,
LinkedList๋ก ๊ตฌํํ ํ๊ฐ ๊ฐ์ฅ ๋์ค์ ์ด๋ผ๊ณ ํ๋ค.
Queue<Integer> q = new LinkedList<>();
import java.util.LinkedList;
public class Main {
public static void main(String[] args) {
LinkedList<String> queue = new LinkedList<String>();
queue.add("ํ๋");
queue.add("๋");
queue.add("์
");
queue.poll();
queue.remove();
queue.clear();
}
}
๋งํฌ๋๋ฆฌ์คํธ๋ฅผ ์ฌ์ฉํด์ ํ (queue) ๊ตฌ์กฐ FIFO ์ ์ ์ ์ถ ๋ฐ์ดํฐ ์ฝ์ , ์ญ์ ๊ธฐ๋ฅ์ ์ฌ์ฉ
add : ํด๋น ํ์ ๋งจ ๋ค์ ์ ๋ฌ๋ ์์๋ฅผ ์ฝ์
offer : ํด๋น ํ์ ๋งจ ๋ค์ ์ ๋ฌ๋ ์์๋ฅผ ์ฝ์
peek : ํด๋น ํ์ ๋งจ ์์ ์๋(์ ์ผ ๋จผ์ ์ ์ฅ๋) ์์๋ฅผ ๋ฐํ
poll : ํด๋น ํ์ ๋งจ ์์ ์๋(์ ์ผ ๋จผ์ ์ ์ฅ๋) ์์๋ฅผ ๋ฐํํ๊ณ , ํด๋น ์์๋ฅผ ํ์์ ์ ๊ฑฐ
remove : ํด๋น ํ์ ๋งจ ์์ ์๋(์ ์ผ ๋จผ์ ์ ์ฅ๋) ์์๋ฅผ ์ ๊ฑฐ
size : ํด๋น ํ์ ํฌ๊ธฐ (์ฌ์ด์ฆ) ๋ฅผ ๊ตฌํฉ๋๋ค
clear : ํด๋น ํ์ ์ ์ฅ๋ ๋ฐ์ดํฐ๋ฅผ ์ ์ฒด ์ญ์
// while ๋ฌธ ์ฌ์ฉํด์ ํด๋น ํ๊ฐ ๋น๋ ๊น์ง ์์ ์ถ๋ ฅ ๊ฐ๋ฅ
while(!queue.isEmpty()) { // ํ์ ๋ฐ์ดํฐ๊ฐ ์์ ๊ฒฝ์ฐ ๋ฐ๋ณต ์ํ ์ค์
System.out.println("poll : " + queue.poll());
}
์๋์ ๊ฐ์ด ArrayList๋ฅผ ์ฌ์ฉํด์๋ ๊ฐ๋จํ๊ฒ Queue๋ฅผ ๊ตฌํํด๋ณผ ์ ์๋ค.
import java.util.ArrayList;
public class App {
public static class _Queue<T> {
private ArrayList<T> queue = new ArrayList<T>();
public void enqueue(T item) {
queue.add(item);
}
public T dequeue() {
if(queue.size() == 0){
return null;
} else {
T x = queue.get(0);
queue.remove(0);
return x;
}
}
}
public static void main(String[] args) throws Exception {
_Queue<Integer> queue = new _Queue<Integer>();
queue.enqueue(1);
queue.enqueue(2);
queue.enqueue(3);
System.out.println(queue.dequeue());
System.out.println(queue.dequeue());
System.out.println(queue.dequeue());
System.out.println(queue.dequeue());
}
}
์ถ๋ ฅ ๊ฒฐ๊ณผ
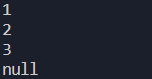
๊ธฐํ ์ฐธ๊ณ ํด๋ณผ ํฌ์คํ : https://go-coding.tistory.com/6
[์๋ฃ๊ตฌ์กฐ] JAVA๋ก ํ(Queue)๊ตฌํ
Queue Queue๋ ์๋ฃ๊ตฌ์กฐ์ ์คํ๊ณผ ๋ฐ๋์ ๊ตฌ์กฐ๋ผ๊ณ ์๊ฐํ๋ฉด ๋๋ค. ํ๋ FIFO(First In First Out)์ ํํ๋ฅผ ๊ฐ์ง๊ฒ ๋ฉ๋๋ค. ๊ฐ์ฅ ๋จผ์ ๋ค์ด์จ ๋ฐ์ดํฐ๊ฐ ๊ฐ์ฅ ๋จผ์ ๋๊ฐ๋ ๊ตฌ์กฐ๋ฅผ ๋งํ๋ค. Enqueue : ํ ๋งจ ๋ค
go-coding.tistory.com
2. Stack
์ฌ์ค ํ์ ๋ก์ง์ ๊ทธ๋๋ก ๊ฐ์ ธ๋ค ์ฐ๋ฉฐ ์คํ์ ๊ตฌํ๋ ์ด๋ ต์ง ์๋ค.
import java.util.ArrayList;
public class App {
public static class _Stack<T> {
private ArrayList<T> stack = new ArrayList<T>();
public void push(T item) {
stack.add(item);
}
public T pop() {
if(stack.size() == 0){
return null;
} else {
T x = stack.get(stack.size()-1);
stack.remove(stack.size()-1);
return x;
}
}
}
public static void main(String[] args) throws Exception {
_Stack<Integer> stack = new _Stack<Integer>();
stack.push(1);
stack.push(2);
stack.push(3);
System.out.println(stack.pop());
System.out.println(stack.pop());
System.out.println(stack.pop());
System.out.println(stack.pop());
}
}
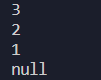